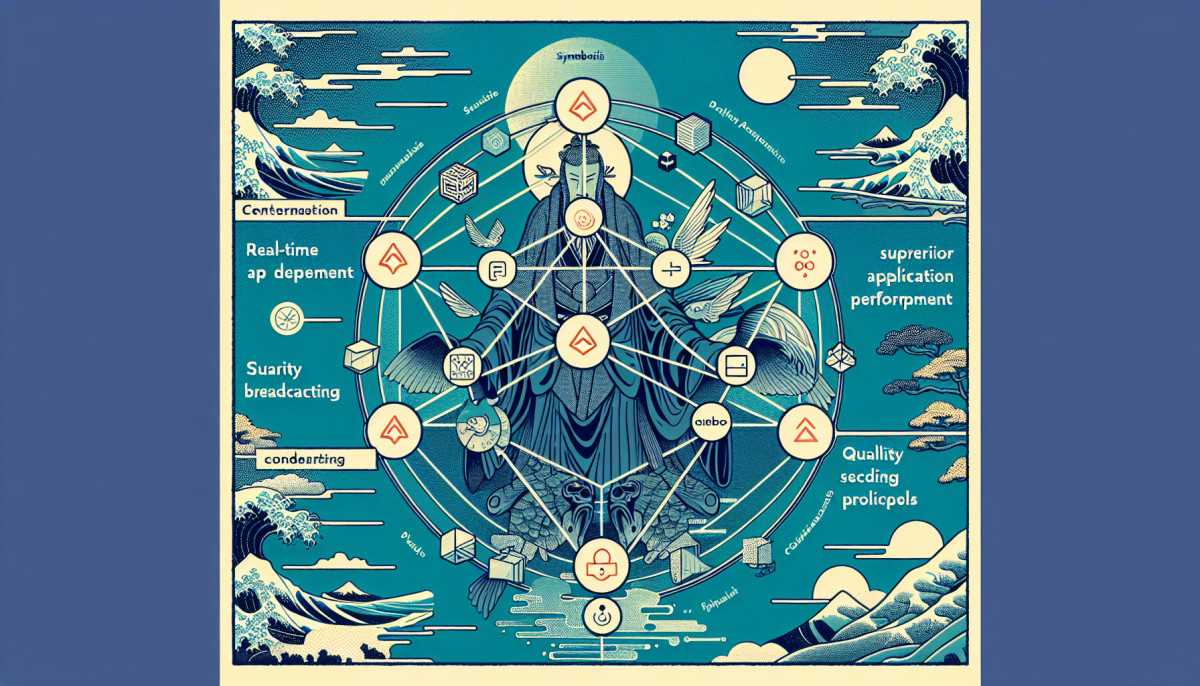
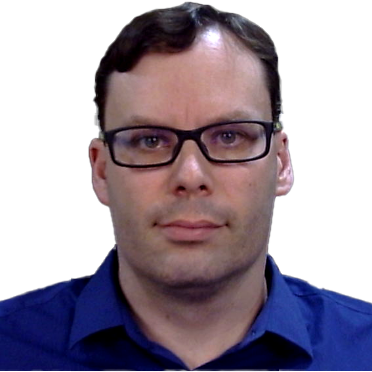
Aug 21, 2023 09:00am
Enhancing user interactions and delivering real-time updates has become a key requirement for contemporary web applications. Among the robust tools meeting this need is Laravel Broadcasting - a potent component of Laravel PHP, with capabilities such as data synchronization, event broadcasting, and real-time communication. Follow along as we delve into the best practices for developing real-time applications with Laravel Broadcasting, employing the prowess of Pusher and Laravel Echo.
Building Strength with Optimized Data Synchronization and Event Broadcasting
Laravel's Broadcasting allows for the efficient transmission of events over WebSockets. The broadcasting of these events keeps users informed of updates in real time, contributing to an interactive and superior user experience.
Streamlining Event Broadcasting
Laravel Broadcasting incorporates three channel types: public, private, and presence channels. Based on the nature and confidentiality of your event data, you can choose the appropriate channel type, thereby optimizing your broadcasting techniques. Here is a simplistic representation of broadcasting an 'OrderShipped' event:
event(new OrderShipped($order));
Event broadcasting is made possible with just a line of code as seen above, where the 'OrderShipped' event is broadcasted to all authenticated users.
Refining Real-Time Data Synchronization
Leverage Laravel's shouldBroadcast
interface to effectively manage real-time data synchronization across multiple users. This interface ensures that any event is queued for broadcasting, optimizing application responses to changes in state and promoting data consistency across users. See the implementation:
class OrderShipped implements ShouldBroadcast
{
//...
}
This code piece demonstrates that the 'OrderShipped' event is queued for broadcasting as soon as the relevant order process is completed. This keeps all users updated with real-time changes.
Scaling and Performance Optimization – Key Pillars of Robust Real-Time Apps
As your Laravel-based real-time app attracts more users, it becomes crucial to implement scalability mechanisms and optimize performance to handle the increased load.
Load Balancing for Scalability
For apps anticipating high user numbers, load balancing ensures consistent performance levels. Use of a load balancer like Nginx, coupled with running your Laravel application across multiple servers, aids in distributing the WebSocket connections equally amongst the servers. Although the specific code depends on your server environment, Laravel's compatibility with leading server software streamlines the setup process.
Leveraging Caching Strategies for Speed
Performance optimization is inextricably linked to effective caching, and Laravel broadcasts offer an integrated API across various caching systems. With Laravel's built-in cache helper and the Cache
facade, developers can store and later retrieve data that would be otherwise expensive to compute or look up. Consider this example:
Cache::put('user:1', $user, 600);
$user = Cache::get('user:1');
This code stores the user details in cache for 600 minutes, thereby reducing database queries each time the user data is fetched, resulting in a faster and more responsive application.
Prioritizing Security in Real-Time Communication
In real-time apps, where data integrity, confidentiality, and security are non-negotiable, Laravel Broadcasting lends itself as a formidable tool against potential security issues.
Emphasizing Safety in Channels and Authentication
Laravel Broadcasting provides an opportunity to establish secure private and presence channels for protecting sensitive data transmission. Middleware-based authentication safeguards ensure that only authorized users emit or listen to events on a given channel.
Broadcast::channel('order.{orderId}', function ($user, $orderId) {
return $user->id === Order::findOrNew($orderId)->user_id;
});
The above code snippet demonstrates the implementation of secure channels, where only the user associated with the order has permission to listen to real-time event updates.
Safeguarding Against Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF)
Preventing security threats like XSS and CSRF is vital to upholding the confidentiality and integrity of real-time apps. Laravel's use of secure Bearer tokens for API authentication and built-in CSRF protection middleware offers significant safeguards against these threats.
Laravel Echo's simple JavaScript syntax also aids in sanitizing user input, thereby preventing potential XSS vulnerabilities:
window.Echo = new Echo({
broadcaster: 'pusher',
key: 'your-pusher-channels-key',
csrfToken: document.head.querySelector('meta[name="csrf-token"]'),
});
This code shows Laravel Echo initializing with a CSRF token, which aids in preventing Cross-Site Request Forgery attacks.
PART IV: Heightening User Experience with Real-Time Features and Interactive Elements
Real-time features and interactive engagement directly influence user experience. The correct implementation of real-time notifications, updates, and Laravel Echo's interactivity can significantly amplify user engagement.
The Power of Real-time Notifications and Updates
Laravel Broadcasting and Laravel Echo simplify the creation and dissemination of real-time notifications and updates. The Laravel notification system is designed to send broadcast type notifications, keeping users informed of significant updates seamlessly.
$user->notify(new OrderShipped($order));
This example shows the notification of an order being shipped sent to a user, ensuring they receive real-time updates on critical activities.
Keeping Users Engaged with Interactive Features
Laravel Echo's listeners enable the application to engage users with dynamic, real-time updates asynchronously. This feature can trigger actions on the client-side which enhances user experiences.
Echo.channel('orders')
.listen('OrderShipped', (e) => {
console.log(e.order.name);
});
In this JavaScript code, Laravel Echo is employed to listen for the 'OrderShipped' event on the 'orders' channel. Interesting and interactive updates like these help in enhancing user engagement.
The Advantages of Laravel Broadcasting for Real-Time Applications
The ability to reflect changes and updates instantly is a driving factor behind why real-time applications are becoming increasingly prominent in today's digital landscape. Tools like Laravel Broadcasting, bolstered by Pusher and Laravel Echo, provide developers with an effective framework for creating powerful real-time applications.
The Increasing Relevance of Real-Time Applications
Building real-time web applications has numerous benefits, including instantaneous updates and enhanced user experience. Laravel PHP, specifically the integration of Laravel Broadcasting and Laravel Echo, offers a structured and efficient environment for real-time application development.
This sample code utilizes Laravel Broadcasting to set up a broadcast channel:
Broadcast::channel('order.{orderId}', function ($user, $orderId) {
return $user->id === Order::findOrNew($orderId)->user_id;
});
An Overview of Laravel Broadcasting, Pusher, and Laravel Echo
In the Laravel PHP framework, Laravel Broadcasting offers a consistent API that allows your application to broadcast events consumed by the client-side. Likewise, as a broadcasting driver, Pusher enables your Laravel applications to communicate in real-time. Meanwhile, Laravel Echo presents a clean, simplistic API for subscribing to channels and listening to event broadcasts.
By employing the best practices highlighted in this blog post, these tools significantly boost your real-time Laravel application's performance and overall user experience.
To summarize, Laravel Broadcasting is an incredibly powerful tool that makes real-time application development a breeze. It equips you as a developer with numerous features such as efficient event broadcasting, real-time data synchronization, security measures, and real-time updates.
Web development continues to evolve rapidly, and technologies like Pusher and Laravel Echo used in conjunction with Laravel Broadcasting ensure you are always producing the best possible applications with efficient, secure communication and optimal user experiences.
If you ever find yourself overwhelmed or in need of an experienced Laravel developer, consider connecting with JerTheDev. With firm knowledge and expertise in Laravel, Laravel Broadcasting, and the associated technologies, JerTheDev is equipped to deliver exceptional Laravel project outcomes, creating real-time applications with advanced features. Feel free to check out the Services page for further details. Happy coding!
Comments
No comments