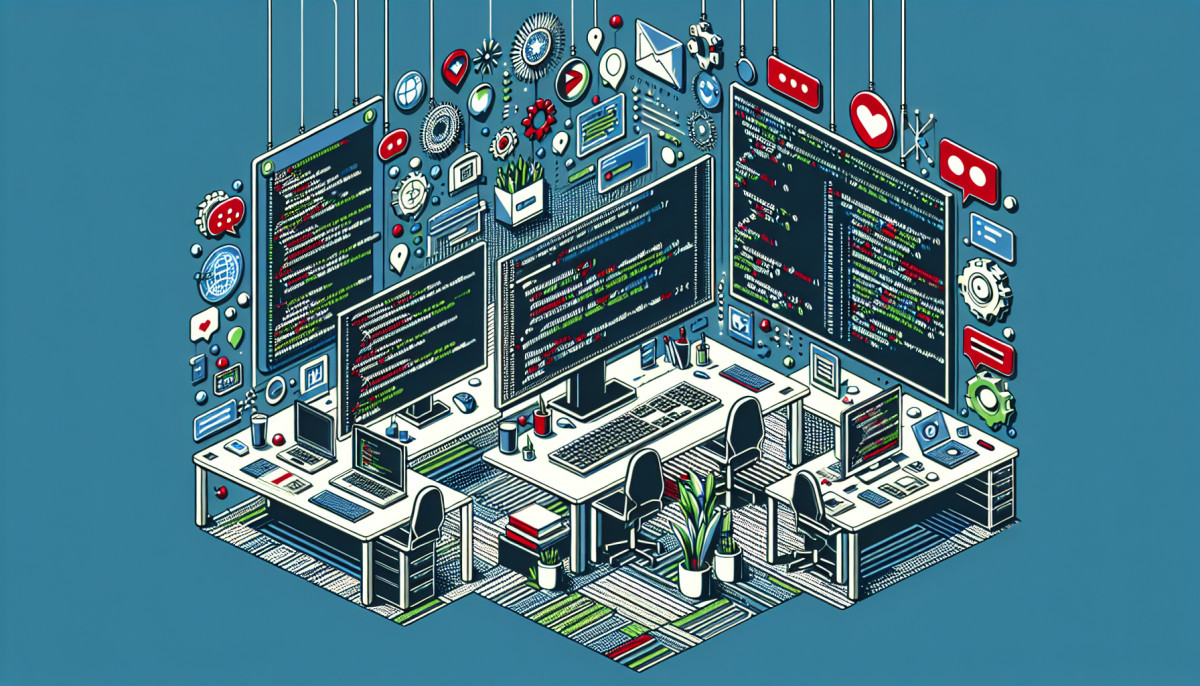
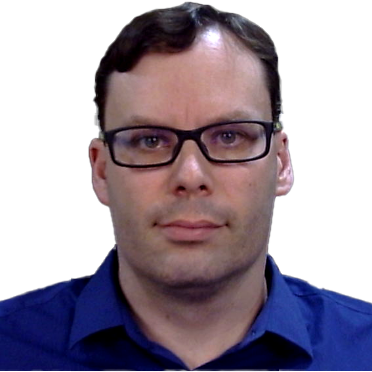
Jan 25, 2024 09:00am
Welcome to another informative piece in our ongoing series about enhancing your WordPress development with APIs. Previously, we discussed the importance of WordPress APIs and their potential uses in detail. This blog post will focus on best practices for developing with WordPress APIs, including vital aspects like security, performance optimization, and standardized practices for seamless WordPress API integration.
Securing Your WordPress API Integrations
When working with WordPress API, maintaining robust security is non-negotiable. Implementing secure connections and establishing strict access control measures can help protect the sensitive data processed by your applications. Let's dive into some tactics to enhance your security measures.
Utilizing HTTPS and Secure Connections
Including an encrypted communication protocol, such as HTTPS, in your WordPress API calls is a crucial step towards a secure data transaction. Using HTTPS ensures your data stays secure during transmission, effectively reducing risks associated with data interception. Here's a generic setup for a secure connection:
fetch('https://example.com/wp-json/wp/v2/posts', {
method: 'GET',
mode: 'cors',
credentials: 'include'
}).then(/*...*/);
This JavaScript code snippet includes HTTPS for secure API connection and incorporates 'credentials: include', enhancing data handling security.
Authorization and Access Control
The WordPress API comes bundled with features for authorizing and controlling data access. Incorporating these features will allow you to define who accesses what data.
$request = new WP_REST_Request('POST', '/wp/v2/posts');
$request->set_header('Authorization', 'Bearer ' . $access_token);
$response = rest_do_request($request);
This example showcases WordPress API authentication— a bearer access token is used, ensuring only authenticated users send requests, thus limiting unauthorized access.
Performance Optimization with WordPress APIs
Optimizing your application's performance is a crucial part of the WordPress development process. Here, we will discuss some strategic practices to enhance your WordPress API’s operational efficiency.
Efficient Data Retrieval and Utilization
Efficiency is key when working with data retrieval. The WordPress API allows parameterized queries, enabling the fetch of specific user-requested data.
fetch('/wp-json/wp/v2/posts?per_page=5&_fields=id,title')
.then(response => response.json())
.then(data => console.log(data))
In this instance, we retrieve only the first five posts, narrowing down to fields that contain the post ID and title, thereby reducing data load and enhancing retrieval speed.
Minimizing API Requests and Payloads
Reducing the frequency of API calls and data size in each call allows faster API responsiveness, and hence better performance. This could be achieved through local storage for data that seldom updates:
let posts;
if(localStorage.getItem('posts')) {
posts = JSON.parse(localStorage.getItem('posts'));
} else {
fetch('/wp-json/wp/v2/posts')
.then(response => response.json())
.then(data => {
posts = data;
localStorage.setItem('posts', JSON.stringify(data));
})
}
console.log(posts);
This code tries fetching posts from local storage first. If unavailable, we make an API request and store it for future use, effectively reducing request frequency and subsequent server load.
WordPress API Best Practices
Following best practices is key to achieving optimal results in WordPress development. Errors are a natural part of development; handling them efficiently can make a substantial difference.
Consistent Error Handling
A well-structured error-handling routine prevents errors from crippling your application. For instance, the WordPress API returns errors in a structured JSON format. Here's a way to handle such errors:
fetch('/wp-json/wp/v2/posts')
.then(response => response.json())
.catch(error => console.error('Error:', error))
.then(response => console.log('Success:', response));
In this example, all error messages from the API are recorded, facilitating timely troubleshooting and issue resolution.
Implementing Data Caching Strategies
Data caching can drastically improve application performance by reducing the number of requests. Here’s how it can be achieved:
<?php
$key = 'my_key';
$data = get_transient($key);
if (false === $data) {
$response = wp_remote_get('https://example.com/wp-json/wp/v2/posts');
$data = wp_remote_retrieve_body( $response );
set_transient($key, $data, HOUR_IN_SECONDS);
}
echo $data;
?>
This code snippet employs transients to store the WP API response, reducing overhead resources and improving performance.
Conclusion
In conclusion, the path to successful WordPress API integration lies in understanding the intricacies of these APIs and adopting time-tested practices. From establishing secure connections to consistent error handling and efficiency in data retrieval, making these techniques your second nature will yield significant improvements in your WordPress development projects.
Remember, the success of your project is directly related to the expertise of your development team. If ever you need the expertise of a skilled Laravel developer, consider hiring JerTheDev! Please visit our Services page to learn all about the benefits of our exquisite web development services. Join us next time as we delve deeper into WordPress APIs, and let's continue learning together with the rest of the content series.
Comments
No comments