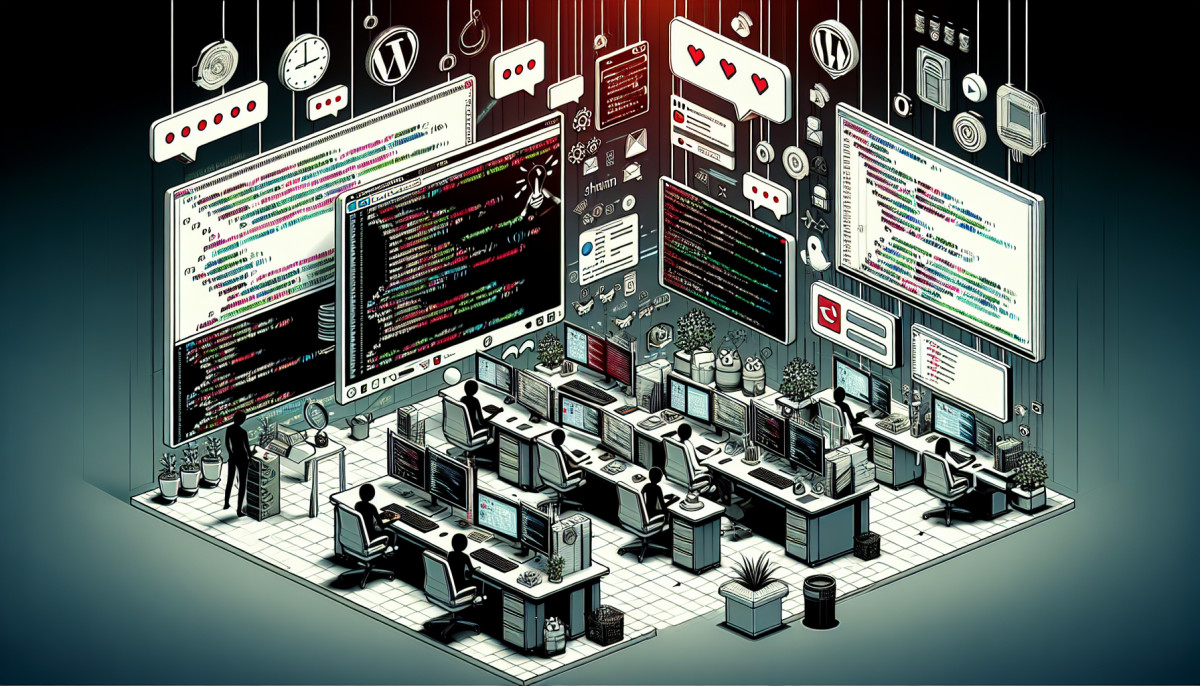
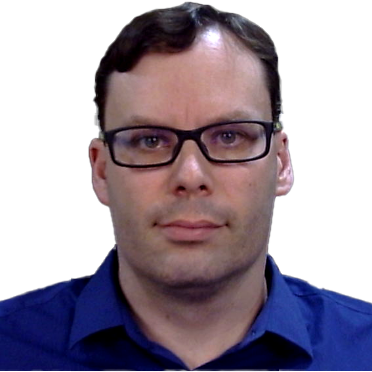
Jan 22, 2024 09:00am
In this ever-evolving world of technology, application programming interfaces (APIs) have emerged as powerful tools for developers. Particularly in WordPress development, understanding how to connect and authenticate with WordPress APIs effectively is indispensable. It provides a secure platform to retrieve and manipulate data, paving the way for dynamic and interactive websites.
This blog post dives deep into the techniques of connecting with WordPress APIs, implementing secure authentication procedures, and the importance of robust API authentication in WordPress. Ensuring secure access, confidentiality and integrity when dealing with WordPress APIs is paramount, hence the focus on these aspects.
Identifying API Endpoints
In any WordPress development project involving APIs, the first step is identifying API endpoints. This refers to specific URLs you interact with to access certain data or functionalities. In WordPress API, these often follow the standard URL format - https://your-wordpress-site.com/wp-json/
.
For instance, if you need to access a list of posts, the corresponding endpoint will be https://your-wordpress-site.com/wp-json/wp/v2/posts
.
$api_url = 'https://your-wordpress-site.com/wp-json/wp/v2/posts';
$response = wp_remote_get($api_url);
With this PHP code snippet, you are ready to retrieve all posts from your WordPress site using the API endpoint.
Connection Methods and Protocols
Identifying the endpoint is just the beginning. Next, establish a secure connection using various methods and protocols. Usually, WordPress development involves using HTTP methods. These include GET, POST, DELETE, and others. It is critical to use HTTPS to ensure secure, encrypted communication. When sending data to the WordPress API, the JSON format is often used due to its readability and compatibility with most programming languages.
Implementing Secure Authentication Procedures
The confidentiality, integrity, and secure access to WordPress API require implementing robust and secure authentication procedures. Developers often use methods like token-based authentication and OAuth mechanisms.
Remarkable Token-based Authentication
Token-based authentication is a popular choice among developers. It entails generating a unique token on the server side and then transferring it to the client side. This token is used for subsequent API requests.
$token = 'your-token';
$headers = array('Authorization' => 'Bearer ' .$token);
$url = 'https://your-wordpress-site.com/wp-json/wp/v2/posts';
$response = wp_remote_get($url, array('headers' => $headers));
The unique token 'Bearer' in the headers ensures secure authentication, as demonstrated in the PHP code above.
Incorporating OAuth and Authorization Mechanisms
OAuth is a standard protocol that allows users to authorize APIs to create and manage their data without needing to share passwords. WordPress supports OAuth 1.0a server plugin.
To use OAuth, start by getting your credentials, including a consumer key and secret. Then request an access token.
$consumer_key = 'your-consumer-key';
$consumer_secret = 'your-consumer-secret';
$headers = array('Authorization' => 'OAuth oauth_consumer_key="'.$consumer_key.'",oauth_token_secret="'.$consumer_secret.'"');
$url = 'https://your-wordpress-site.com/wp-json/wp/v2/posts';
$response = wp_remote_get($url, array('headers' => $headers));
As shown in this command, OAuth credentials are applied to authenticate WordPress APIs.
Token-based and OAuth techniques provide secure ways to authenticate your WordPress API connections. Choosing the right method is essential, as it needs to suit your application's needs and security requirements.
Understanding API Authentication in WordPress
Effective API authentication cannot be overemphasized when establishing secure connections and ensuring authorized access to WordPress APIs. API authentication enables developers to interact securely with the vast tools and data of WordPress.
Overview of Authentication Methods
There are many ways to authenticate and connect with WordPress APIs, but the token-based one is most common. It involves programmers exchanging a secure token for proof of identity.
$api_url = 'https://your-wordpress-site.com/wp-json/';
$token = 'your-token';
$headers = array('Authorization' => 'Bearer ' . $token);
$response = wp_remote_get($api_url, array('headers' => $headers));
This code snippet demonstrates the simple implementation of token-based authentication in WordPress API.
Importance of Secure Authentication
Secure API authentication is more than crucial - it's mandatory. It helps you prevent unauthorized access, safeguarding users and developers. Without it, your WordPress development projects could be prone to data breaches or misuse, thus compromising data integrity and confidentiality.
Understanding and employing secure authentication methods is integral to any WordPress development process. Embracing these secure protocols allows you to unlock the full potential of what the WordPress API suite can do while ensuring all transactions and information exchanges remain secure.
In summary, both ensuring successful connections to WordPress API endpoints and employing robust, secure authentication methods are crucial components of WordPress development. This comprehensive understanding will assist programmers in building more effective, interactive, and secure websites or applications with WordPress.
Are you looking for a Laravel developer for your next project? Consider hiring JerTheDev. Check out the Services page and feel free to reach out. Drive your WordPress development project to success with professional developers who have a thorough understanding of connecting and authenticating with WordPress APIs!
Comments
No comments