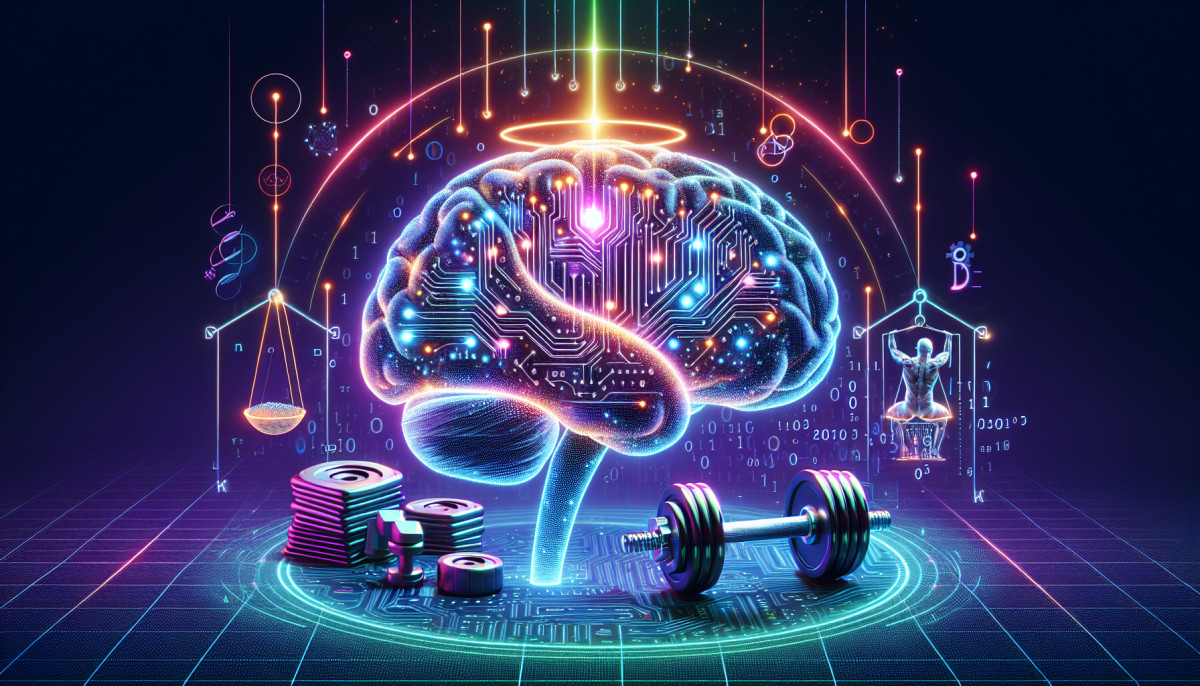
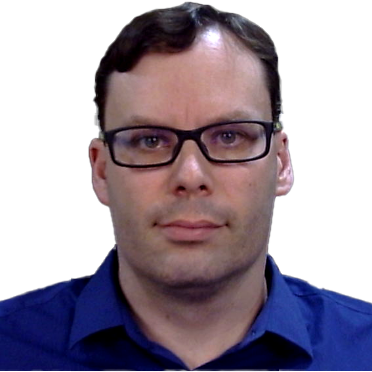
Nov 20, 2023 09:00am
Artificial Intelligence programming is a fascinating field that is challenged by the versatility of programming languages and the complexity of AI methodologies. Today, we dive into the realm of AI programming using Python through OpenAI Gym - an exceptional platform that not only aids in honing your Python skills but also serves as an ideal testing ground for sophisticated AI algorithms.
Reinforcement Learning Demystified
Reinforcement Learning is a remarkable offshoot of Artificial Intelligence that thrives on the concept of learning through feedback - essentially, the law of cause and effect, or reward and punishment. An exemplary Pythonic representation of reinforcement learning would be this piece of pseudo-code:
## Pseudo-code for a simple reinforcement learning algorithm
Initialize state S
Repeat (for each step of the episode):
Choose action A from state S using a policy derived from Q
Take action A
Get reward R and next state S'
Update Q(S,A) <- Q(S,A) + alpha*[R + discount_factor*max Q(S’,a) - Q(S,A)]
S <- S'
until S is terminal
This basic reinforcement learning algorithm exhibits the underlying concept of strategy optimization via iterative trial-and-error, taking cues from reward feedback.
Introducing OpenAI Gym in AI Programming
OpenAI Gym is a brilliant amalgamation of different reinforcement learning tasks. It provides a standardized, robust interface which simplifies the process of developing and comparing reinforcement learning algorithms in Python. Not to mention, it is an excellent platform for reproducing these algorithms for test and future use. Here is a simple Python code representation of how to set up the AI task in OpenAI Gym:
import gym
env = gym.make('CartPole-v0')
observation = env.reset()
for _ in range(1000):
env.render()
action = env.action_space.sample() # your agent here
observation, reward, done, info = env.step(action)
This Python code constructs a simple game-playing agent using OpenAI Gym, thereby perfectly demonstrating the utility of OpenAI Gym in AI programming.
Enriching Your AI Programming Skills with OpenAI Gym
With OpenAI Gym, discover how you can implement reinforcement learning algorithms range from the simplest reward-based systems to more intricate game-playing agents. Moreover, build exquisite AI applications within Python, deriving the foundational reinforcement learning algorithms.
Building Reinforcement Learning Algorithms
Creating reinforcement learning algorithms using OpenAI Gym is an intuitive process. Below is an example of how you can train a Q-learning agent in the environmental scenario 'CartPole':
import gym
import numpy as np
env = gym.make('CartPole-v0')
Q = np.zeros([env.observation_space.n, env.action_space.n])
for episode in range(1,1001):
state = env.reset()
done = False
while not done:
action = np.argmax(Q[state])
state2, reward, done, info = env.step(action)
Q[state, action] += 0.5 * (reward + np.max(Q[state2]) - Q[state, action])
state = state2
This algorithm gives you a glimpse into training a reinforcement learning model via Q-learning in an OpenAI Gym environment.
Adventuring into AI Applications with OpenAI Gym
Once you have a solid grasp of fundamental reinforcement learning algorithms, you can start extending the boundaries of AI applications. Use OpenAI Gym to build intricate AI applications such as game-playing AI for video games, intelligent chatbots, advanced trades systems, and much more. They can be built within the Python environment, employing the principles learnt from the gym scenarios.
Even if the predefined environments within the OpenAI Gym do not suit your needs, remember, it provides you the freedom to create your own custom environments. So, irrespective of the diversity in your requirements, rest assured, OpenAI Gym, combined with Python, provides you a one-stop solution for AI programming.
Defining Unique AI Scenarios with OpenAI Gym
Let’s further enhance our understanding of OpenAI Gym, by learning how to define and create custom environments. Understand and master the ability of creating unique AI situations to test your advanced algorithms.
Establishing Custom Environments
By creating your custom environments, you can define specific observations, actions, and measures of rewards. Such a customized approach lends focus to specific learning objectives and project needs. Here's a standard layout for creating a custom environment in Python using OpenAI Gym:
import gym
from gym import spaces
class CustomEnv(gym.Env):
def __init__(self):
super(CustomEnv, self).__init__()
# Define action and observation space
self.action_space = spaces.Discrete(N_DISCRETE_ACTIONS)
self.observation_space = spaces.Box(low=-180, high=180, shape=(3,))
def step(self, action):
pass # Implement your step logic here
def reset(self):
pass
This code shows an example of a CustomEnv
inheriting from gym.Env
, and how to customize key methods like __init__()
, step()
, and reset()
.
Designing Challenges
Once you’ve laid down the boundaries of your custom environment, it's time to introduce complexities or gaming scenarios for the AI agent. These modifications keep your coding skills sharp and intuitive. Here's an example, where various levels of difficulties are introduced within a game playing environment.
def step(self, action):
if self.level == 'hard':
self.speed = 2.0
elif self.level == 'medium':
self.speed = 1.0
else:
self.speed = 0.5
# Other game logic here
This showcases the flexibility of OpenAI Gym and Python, where you can introduce diverse complexities, thereby enriching your current project goals.
Time to Get Your Hands Dirty with OpenAI Gym in Python
Buckle up to dive into this thrilling journey into the world of OpenAI Gym. Kick-start this exciting adventure by understanding the navigation process and the key concepts involved in using this extensive toolkit for AI programming in Python.
OpenAI Gym Installation Guide
Integrating OpenAI Gym into your Python workspace is a breeze. Start with a Python installation followed by pip. Next, use the following command to install OpenAI Gym:
pip install gym
pip install gym[atari]
Verify the successful installation by importing the gym library in Python as shown below:
import gym
print(gym.__version__)
Decoding the OpenAI Lingo
Work on understanding OpenAI Gym's core concepts. Each simulation in Gym revolves around an 'environment' upon which an AI task takes place. Every environment encapsulates specific 'actions' performed by the AI agent. 'Observations' give feedback to the agent about the environment after each action.
You can interact with the environment following these commands:
env = gym.make('CartPole-v0') # create an environment
env.reset() # reset the environment
env.step(env.action_space.sample()) # take a random action
Conclusion
OpenAI Gym combined with Python, forms a versatile platform to experiment, learn, create, and reproduce AI algorithms and applications. This dynamic duo of OpenAI Gym and Python makes the journey into the world of AI programming an exciting adventure. Whether you are a beginner or an experienced programmer, OpenAI Gym has something for everyone.
Though challenging, the world of AI programming is incredibly exciting, and it promises immense professional and personal satisfaction. If you ever consider taking your programming skills to the next level by building bigger, more complex projects but need a helping hand, consider enlisting the support of a professional Laravel developer. Feel free to explore how Jeremy Fall, also known as JerTheDev, can assist you on your journey. To know more, visit our Services page.
Just remember, as Neils Bohr once said, "An expert is a person who has made all the mistakes that can be made in a very narrow field." Making mistakes and learning from them is part of the journey. Happy coding!
Comments
No comments