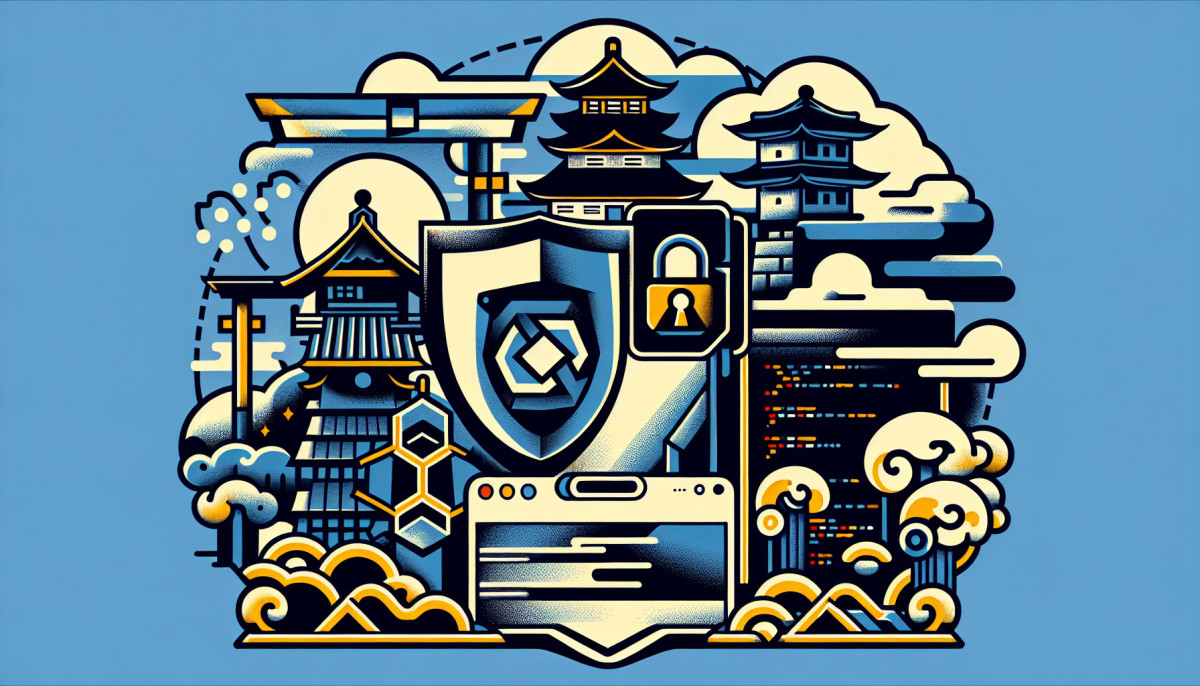
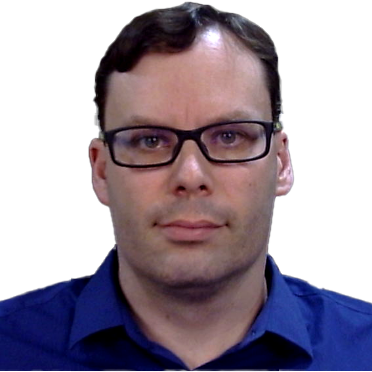
Nov 09, 2023 09:00am
In the realm of web development, security is paramount. One such pillar of web security is Role-Based Access Control (RBAC). Leveraging this security technique in PHP frameworks such as Laravel establishes a guard for your application, delineating precisely who gets to interact with what in your platform, ensuring your Laravel security is top-tier. In this comprehensive guide, we will discuss, in detail, the essential concepts, components, and the practical implementation of RBAC in Laravel, that will help reinforce your Laravel PHP application's safeguarding capabilities.
The purpose of this guide is to empower you as a Laravel developer to implement robust and efficient access control systems in your applications. If you're considering hiring a Laravel developer, take note of JerTheDev's proven expertise and be sure to check out the services page.
Understanding Role-Based Access Control (RBAC)
Applying RBAC in Laravel essentially births permission levels through roles like sentinels guarding a city fortress. Each role possesses a defined set of permissions determining which sectors of the application it can access, similar to how our sentinel would have designated patrol areas.
Consider a Laravel-developed content management system; you may have differentiated roles such as 'Editor', 'Author', and 'Admin', each with contrasting system access levels, distinctly defined through the intimate relationship between roles and permissions. A simplified depiction following Laravel code example here:
class Role extends Model
{
public function permissions()
{
return $this->belongsToMany(Permission::class);
}
}
class Permission extends Model
{
public function roles()
{
return $this->belongsToMany(Role::class);
}
}
Access Control Lists (ACLs) are another pivotal component in your Laravel security arsenal. An ACL is a mechanism mapping roles and permissions to users, effectively managing access rights. Laravel incorporates policies and gates within its ACL environment to substantiate permissions before permitting user interaction.
Gate::define('edit-articles', function ($user) {
return $user->hasRole('Editor');
});
In this Laravel code snippet, the 'edit-articles' permission is tethered to 'Editor' allowing anyone holding the 'Editor' role to edit articles, highlighting the Laravel ACL's elegance.
Optimizing Laravel Security with RBAC
Efficient Laravel security isn't merely about successful implementation; it also entails constant evolution. Monitoring access activities, reviewing, and refining access policies are integral measures to stay ahead of any potential security vulnerabilites.
Auditing and Monitoring access activities open a window into your application's operations enabling you to perceive and address potential security issues. Laravel PHP furnishes us with built-in logging capabilities such as recording administrative modifications, data manipulations, and tracking login attempts.
use Illuminate\Support\Facades\Auth;
...
public function handle(Request $request, Closure $next)
{
activity()
->causedBy(Auth::user())
->log('User logged in');
return $next($request);
}
With Laravel's built-in logging functionality, you can track any user's interaction with your application, as demonstrated by recording a user's login activity in the above Laravel PHP code example.
Applications evolve, and as they do, their access policies need to follow suit. A dynamic Laravel security protocol involves regularly reviewing and updating access permissions. Laravel provides the capability to update these as the application grows, as depicted in the following PHP change:
$role = Role::findByName('Editor');
$role->syncPermissions(['edit articles', 'publish articles']);
The above piece of Laravel PHP code details how you can seamlessly update the permissions for an existing role, ensuring your Laravel security remains robust and adaptable to changing requirements and challenges.
Implementing RBAC in Laravel
Laravel PHP comes with built-in mechanisms enabling you to form durable, trustworthy, and seamless role-based access control systems, reinforcing Laravel security immeasurably.
To configure role-based permissions in Laravel, all you need is to resort to Laravel's intrinsic methods. An 'Admin' granted the role to 'delete users' would look something like the following Laravel code:
$role = Role::findByName('Admin');
$permission = Permission::findByName('delete users');
$role->givePermissionTo($permission);
Laravel also simplifies user role management and associated access levels. Assigning an 'Admin' role to a user, and later revoking it, becomes as simple as recording a dance step:
$user = User::findByEmail('user@email.com');
$user->assignRole('Admin');
...
$user->removeRole('Admin');
Therefore, it becomes necessary to systematically approach Role-Based Access Control to strengthen the security of your Laravel PHP application substantially. Laravel facilitates every Laravel developer with intricate control over each user role's permissions, empowering you to employ rigorous security measures for your application.
To conclude, the complexity of Laravel security can be intimidating, and implementing it requires a workable level of expertise. If you need further assistance in propelling your Laravel project or up your Laravel security game, consider partnering with a Laravel developer like JerTheDev. Do check out our Services page to learn more about how we can help fortify your Laravel applications!
Comments
No comments