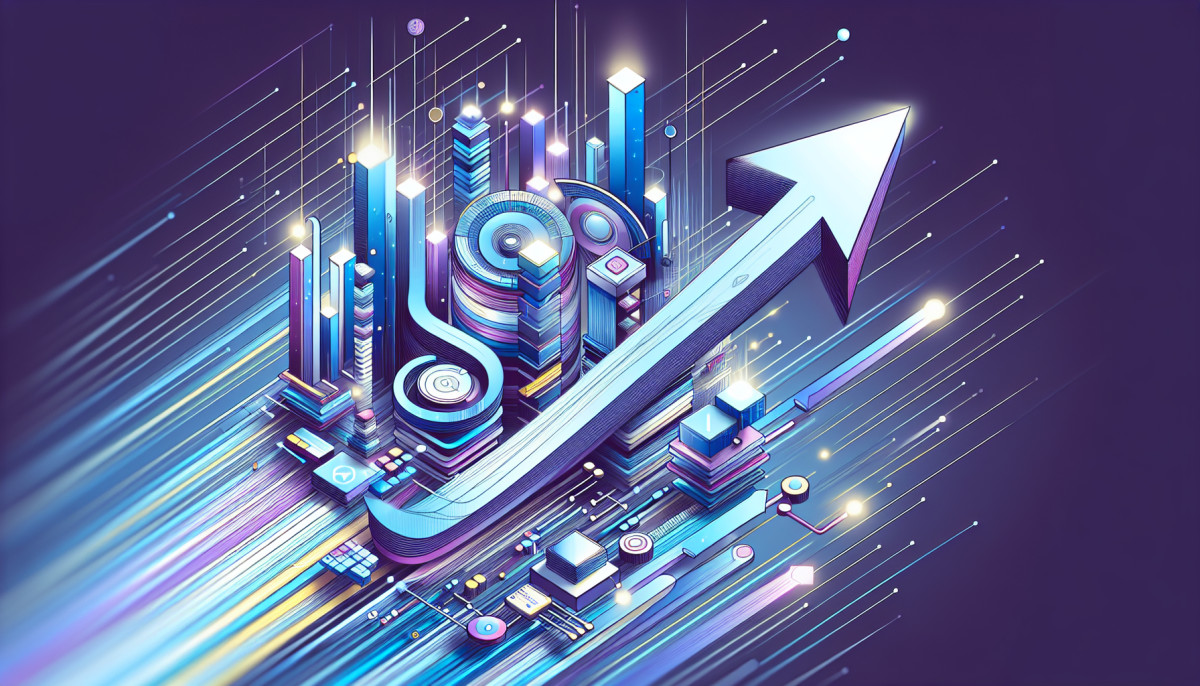
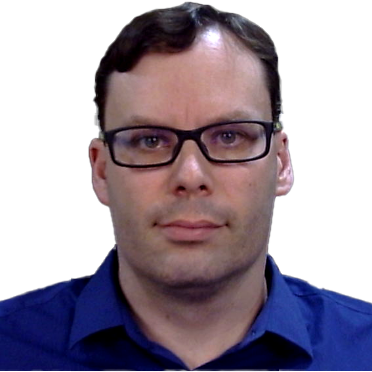
Aug 31, 2023 09:00am
As a Laravel developer, you already know that Laravel PHP offers an extremely efficient, feature-packed framework for developing web applications. One important element in Laravel's toolbox is Laravel Collections, which provides an intuitive, flexible interface for dealing with array data. But are you harnessing the full power of Laravel Collections? This guide will offer insights on how to maximize efficiency and performance with Laravel Collections. Our focus will be on advanced techniques and best practices that you can use to take your Laravel applications to the next level.
Unlocking the Power of Efficiency and Performance
The Laravel Collections framework is already robust and capable out of the box, but with the right techniques, you can enhance its capabilities exponentially to serve your specific needs. One strategy you must cultivate for efficiency maximization is the utilization of Lazy Collections.
The Magic of Lazy Collections
Lazy Collections, a feature introduced in Laravel 6.0, loads data as needed rather than all at once. This allows it to process large datasets while conserving memory. By loading only a small part of the data set into memory at a time, it allows for smoother processing of enormous data loads, a significant leap in PHP development.
use App\Models\User;
use Illuminate\Support\LazyCollection;
LazyCollection::make(function () {
$file = fopen(storage_path('app/users.csv'), 'r');
while ($data = fgetcsv($file)) {
yield $data;
}
})->chunk(200)->map(function ($users) {
return (new User)->fill([
'name' => $users[0],
'email' => $users[1],
]);
});
This code leverages the 'make' function, which yields user data from a CSV file. It then chunks the data into manageable bits and maps out user instances. The overall effect? Powerful on-demand data processing that won't compromise system performance.
Efficiency Through Deferred Execution
Another powerful concept in Laravel Collections is deferred execution. Rather than processing data immediately, Laravel Collections can store certain operations until the results are actually needed. This efficient method adds another layer of performance optimization, conserving resources, and maintaining system responsiveness even in the face of large data sets.
Advanced Performance with Laravel Collections
Laravel Collections rise to the top in terms of providing customization options that suit specific application needs while simultaneously pushing the boundaries of optimization.
Defining Custom Collection Methods
While Laravel Collections already offer a wide breadth of built-in methods, Laravel makes it simple for developers to define custom collection methods using the macro
function. This flexibility allows you to craft methods that specifically cater to the requirements of your application while enhancing its functionality. Laravel Collections also offer a range of advanced optimization techniques like higher-order messages and the spread operator for optimized performance.
Enhancing Performance with Best Practices
To extract the maximum performance out of Laravel Collections, it's crucial to maintain optimized queries and ensure efficient resource utilization. The pluck
method allows for faster data retrieval by fetching only needed values from a collection, resulting in improved memory usage and system efficiency.
Collection methods like map
, reduce
, and filter
also come in handy, aiding in refining data subsets, carrying out specific computations, and executing particular conditions for data extraction. These techniques contribute to a more efficient resource utilization, benefiting the overall system performance.
Laravel Collections: Stepping Up Your Laravel Game
In essence, Laravel Collections offer Laravel developers with an efficient paradigm to handle array data structures. Through strong concepts and powerful functionalities, Laravel Collections empower Laravel developers to boost code efficiency and enhance application performance.
If my guide has excited you about the possibilities and advantages of Laravel Collections, why not take the next step? If you're in need of an experienced Laravel developer or require more extensive assistance, consider hiring JerTheDev. Feel free to check out my Services page. Let's unlock the true potential of Laravel Collections together!
Comments
No comments