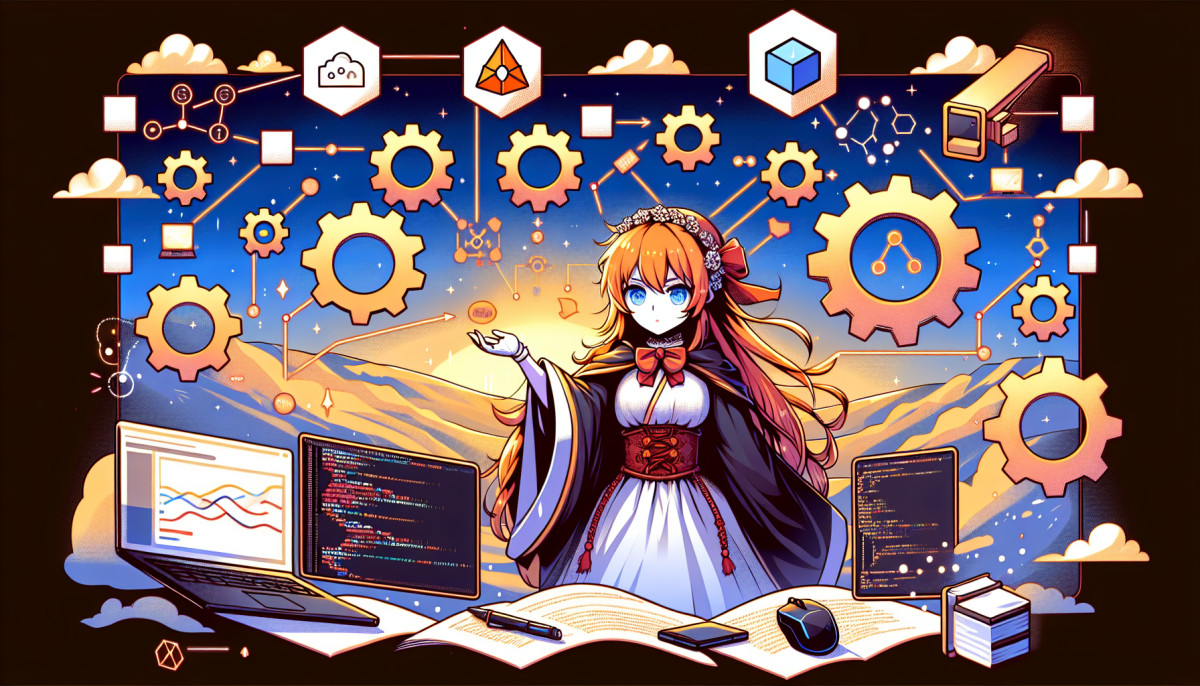
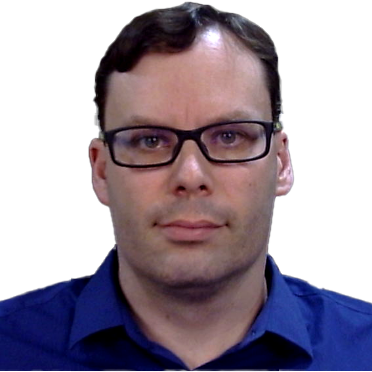
Aug 28, 2023 09:00am
In this world of digital modernization, creating efficient web applications has become a crucial factor for success. A solid knowledge of application development methodologies is paramount, and Laravel, a comprehensive PHP framework, meets these requirements. Known for its clean syntax and robust functionality, the Laravel PHP framework has become a go-to for many developers worldwide. The accessibility it offers and its elegant way of dealing with complex programming tasks are truly impressive. Laravel collections, the subject of our blog post today, take these merits even further.
Introduction to Laravel Collections
Laravel collections add a layer of functionality to PHP arrays, transforming them into supremely malleable objects. They offer tools and methods that programmers, especially a dedicated Laravel developer, can utilize for effective and hassle-free data handling within Laravel applications.
$collection = collect(['Taylor', 'Abigail', 'James']);
As seen above, a Laravel Collection can be created from a basic PHP array. Doing this arms developers with several additional functionalities that Laravel collections offer. A core advantage of Laravel Collections is the enhancement of PHP array functions. Meaning, when working with Laravel Collections, array functions get a significant power-up, enabling one to use them in an object-oriented manner.
$collection = collect(['Taylor', 'Abigail', 'James']);
$first = $collection->first();
Here, we see the method to fetch the first record. The $first
variable will return 'Taylor', and undertaking this wouldn't be any simpler than with Laravel Collections.
Core Collection Methods
Having understood the basics, let's look at some core Laravel Collection methods that facilitate data filtering, transformation, sorting, and reduction.
Filtering and Transformation
With a wide array of filtering and transformation capabilities, Laravel Collections aid developers in deftly handling data. A prime example would be the Filter method. This function allows developers to use specific conditions to filter data in collections.
$collection = collect([1, 2, 3, 4, 5, 6, 7, 8, 9]);
$filtered = $collection->filter(function ($value, $key) {
return $value > 5;
});
In this illustration, the $filtered
will offer a new Laravel collection containing items greater than 5.
Sorting and Reducing Data
Laravel Collections also simplifies the sorting and reduction of data. Consider the Sort method:
$collection = collect([5, 3, 1, 2, 4]);
$sorted = $collection->sort();
This Sort expression will return $sorted
with a new sorted collection (1, 2, 3, 4, 5). Likewise, collections allows retrieval of a single value from the collection through the Reduce method. By understanding these features, developers will find Laravel Collections a revolutionary tool for seamlessly manipulating data in Laravel PHP.
Techniques for Optimizing Data Manipulation
Efficient web app development requires proficient data handling techniques. In Laravel Collections, the key is to find effective methods for enhancing the performance of your application.
Performance Optimization
A developer can improve the efficiency through eager loading which reduces SQL queries when retrieving data using Laravel's ORM Eloquent. It speeds up the data fetching process by retrieving related objects at once:
$users = App\Models\User::with('orders')->get();
In this example, the with
function performs eager loading of 'orders', which enhances the app's performance by limiting the required SQL query calls.
Effective Data Management
Strategizing for effective data usage through Laravel Collections is vital. Laravel's features like eager loading, mass assignment, and the fluent builder pattern, all contribute to effective and efficient data manipulation.
$flight = App\Models\Flight::create(['name' => 'Flight 10']);
The above occurrence demonstrates the mass assignment method, an effective practice in Laravel collections. Combined wisely, these functionalities enable a Laravel developer to craft a high-performing, streamlined application with finesse.
Advanced Data Manipulation
Now that we've covered the basics and core methods available in Laravel collections- a competent Laravel Developer can use the framework to its full potential. Laravel Collections allow us to perform advanced data transformation and customize the behavior of the collections.
Customization Methods
Customizing your collection behavior can enhance its utility and control in Laravel PHP. Developers can extend Laravel collections by adding macros:
use Illuminate\Support\Collection;
Collection::macro('toUpper', function () {
return $this->map(function ($value) {
return strtoupper($value);
});
});
$collection = collect(['first', 'second']);
$upper = $collection->toUpper();
Above, a 'toUpper' macro is added to the Collection class enabling transformation of collection items into uppercase.
Advanced Data Transformation
Advanced transformations add flexibility in dealing with complex data.
$collection = collect([
['name' => 'John', 'profession' => 'developer'],
['name' => 'Jane', 'profession' => 'designer'],
]);
$flattened = $collection->flatMap(function ($values) {
return array_map('strtoupper', $values);
});
The $flattened
collection contains all transformed values in uppercase.
Once mastered, Laravel Collections unravel advanced transformations that add tremendous value to your Laravel development skillset, empowering you to handle complex projects seamlessly.
In Conclusion
Laravel Collections, with their feature-rich toolset, enhance Laravel PHP’s capacity for data manipulation to an impressive degree. They wrap common PHP array functionalities into a more user-friendly, object-oriented package, providing developers with an efficient means to execute intricate operations - from filtering and transforming data to optimizing performance, from managing data effectively to carrying out intricate transformations, and even customizing application behavior according to one's needs.
Whether you’re a seasoned Laravel Developer or a beginner, Laravel Collections is a powerful tool to have in your repertoire, owing to its ability to simplify data manipulation within Laravel PHP applications.
Extend Your Skills
Don’t stop now; Laravel has much more to offer! If you are considering hiring a Laravel developer, look no further. JerTheDev offers unparalled development services and is always ready to take on new challenges. Do consider hiring JerTheDev for your next Laravel project, and check out the Services page for more information.
Stay tuned for more insightful posts on Laravel Collections as we unfold its power in our upcoming articles from the series - Harnessing Efficiency and Performance with Laravel Collections: An Insider's Guide. Happy coding!
Comments
No comments