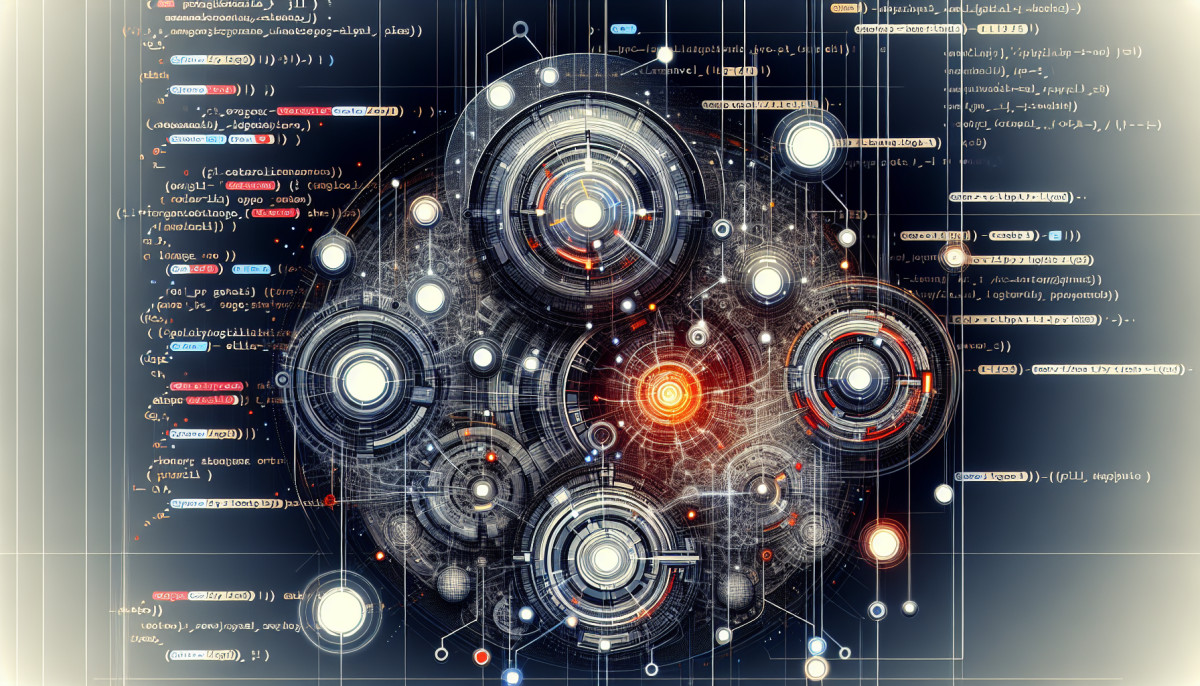
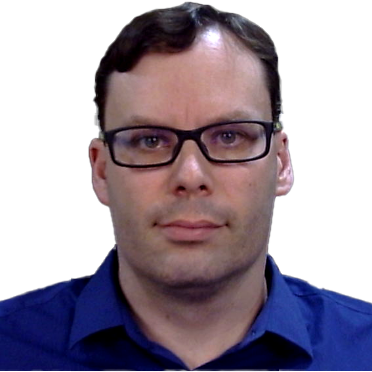
Sep 04, 2023 09:00am
Today, we're taking a deep dive into Laravel PHP’s event-driven architecture and how it enables developers to create highly scalable, efficient applications. We'll be breaking down the core concepts, examining the benefits, and outlining the implementation process step-by-step. By the end of this post, you should have a solid understanding of Laravel events, how they help structure your code, and how to implement them to your advantage. This post aims to empower you, the Laravel developer, to thrive in the modern software development landscape.
Laravel and Event-Driven Architecture: A Brief Overview
At its core, event-driven architecture is all about events – occurrences that matter to the system. In a Laravel PHP context, these could range from a new user registering, to a product being added to a shopping cart, to a transaction being completed. Each of these actions triggers specific events that can induce other actions or even chains of actions.
This sort of interaction can be illustrated with a simple Laravel command, such as event(new NewUserRegisteredEvent($user));
which shows an example of triggering an event in Laravel when a new user registers.
The event-driven approach enables Laravel developers to write cleaner, more organized code, improving maintainability and scalability. It encapsulates logic within individual event listeners and dispatchers, which facilitates parallel execution of multiple tasks or services - a great advantage for scalability. If you're a Laravel developer looking for a smarter way to organize your application and improve performance, Laravel events are a game-changer.
How to Implement Laravel Events for Optimal Performance
Leveraging Laravel's event-driven architecture requires understanding three core steps: Defining events and listeners, registering event-listener associations, and dispatching events.
Defining Events and Listeners
Events and their corresponding listeners need to be defined in Laravel. This is done in the app/Events
and app/Listeners
directories respectively. The Laravel command lines for this might look something like php artisan make:event NewUserRegisteredEvent
and php artisan make:listener SendWelcomeEmailListener --event=NewUserRegisteredEvent
.
Registering Events and Listeners
This step involves tying together the events and listeners. Laravel does this inside the EventServiceProvider
, located within the app/Providers
directory. An example could look like protected $listen = ['App\Events\NewUserRegisteredEvent' => [ 'App\Listeners\SendWelcomeEmailListener', ], ];
.
Dispatching Events
Once events and listeners are defined and registered, Laravel triggers, or dispatches, the events. Laravel takes care of this automatically when an event is dispatched through the event
helper function.
By now, you should have an operational event-driven architecture in your Laravel application. But don't just stop there - let's explore event handlers and dispatching next.
Event Handling and Dispatching: The Power Behind Laravel Events
Within Laravel's event-driven architecture, event handlers, or listeners, and the process of event dispatching stand at the core. They are what allow your applications to respond dynamically to events.
Handling Events
Listeners do the heavy lifting when it comes to reacting to events in Laravel. They receive the event data when an event is dispatched and start a programmed response.
Dispatching Custom Events
Custom events allow developers to set up communication lines between different parts of a Laravel application. By dispatching a custom event, the programmer is telling Laravel to let associated listeners know that an event has occurred and that they should react accordingly.
Laravel also allows control over the order of listeners and can even stop the propagation of an event if a developer needs to do so. Returning a false from a listener will halt the calling of any remaining listeners, adding an extra level of control and customization to Laravel applications.
The Core Components of Laravel's Event-Driven Architecture
Three key components make up Laravel's event-driven architecture: events, listeners, and the event dispatcher.
At their most basic, events are signals telling Laravel that an action occurs. They contain information about the occurrence that can be used in the application. Each listener is tied to a specific event and defines how the application should react when that event occurs.
The dispatcher acts as the central hub, which is responsible for alerting all listeners when an event is fired. Together, these components form the backbone of Laravel's event-driven structure and are crucial to fully understand for any Laravel developer.
Embracing Laravel’s Event-Driven Architecture
Laravel's event-driven architecture offers scalability, efficiency, and dynamic responsiveness to web applications, making it an essential tool for every Laravel developer. Couple this with the robustness and flexibility of Laravel, and you have a powerful toolset enabling you to deliver enhanced user experiences and efficient development processes.
Event-driven architecture is gaining traction and is expected to play a significant role in future trends in web application development. As real-time event processing becomes more common, Laravel’s event-driven architecture is perfectly placed to take advantage.
By understanding and implementing Laravel events, developers can craft applications that are not only more efficient and organized but are also more flexible, dynamic, and capable of handling more demand. This improves user experience and gives developers the tools to meet increasing demands.
Conclusion: The Power of Laravel PHP’s Event-Driven Architecture
As Laravel PHP continues to grow in popularity among professional developers, those who can effectively harness the capabilities of its event-driven architecture will stand at the forefront of application development. One needs no better argument for diving into Laravel events and fully understanding how to implement them in a Laravel PHP project.
If learning all of this seems daunting, you might want to consider enlisting the help of an experienced Laravel developer like JerTheDev to ensure your application is built to highest standards using modern best practice. To learn more about the services that JerTheDev offers, please check out the Services page. By partnering with an expert who understands the power of Laravel's event-driven architecture, you can guarantee that your application is in safe, competent hands.
You've embarked on the journey of understanding the basics of Laravel's event-driven architecture. Continue this learning by delving into the rest of the series – "Implementing Event-driven Architecture in Laravel: Best Practices and Tips" and "Unleashing the Power of Event-driven Architecture in Laravel: Real-world Examples and Case Studies." Event-driven architecture is not just a trend; it's the future. So, let's embrace it and move towards a more scalable and efficient world of Laravel PHP application development.
Comments
No comments