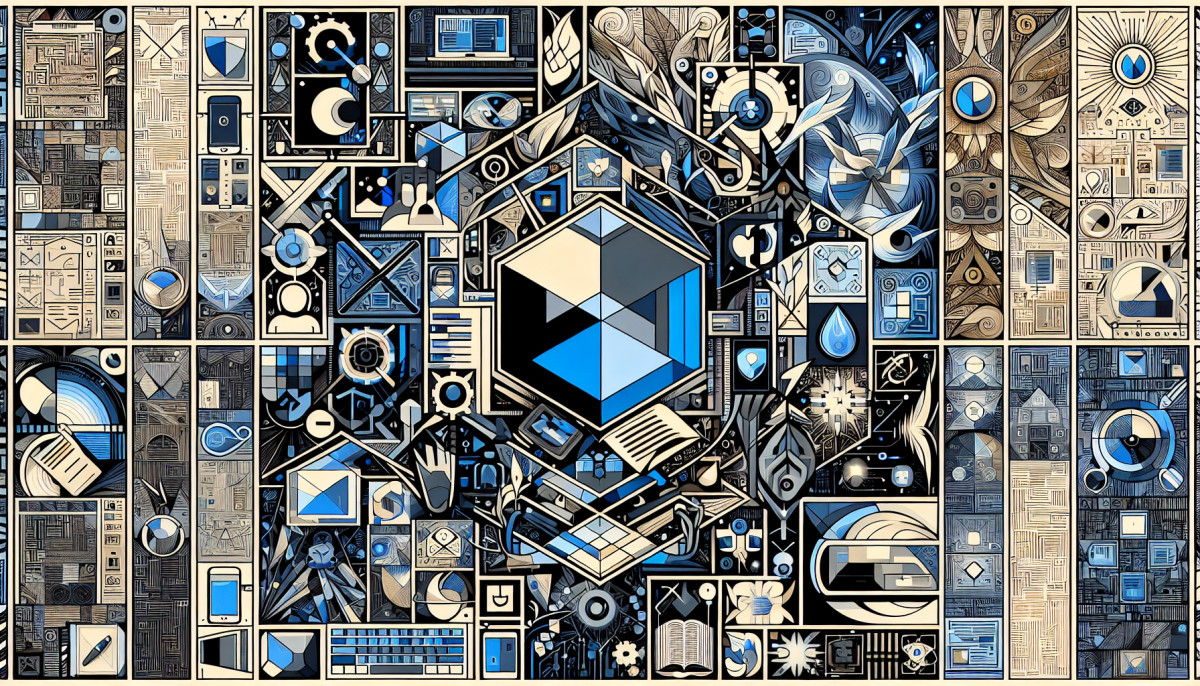
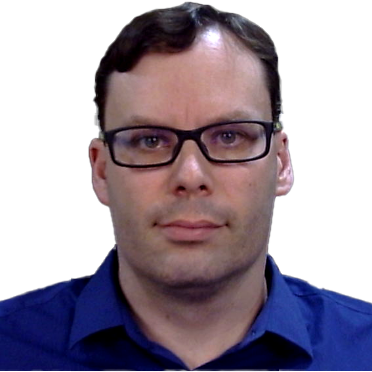
Aug 24, 2023 09:00am
The Laravel PHP framework has long been a cornerstone in the world of web development, offering an extensive database of creative, efficient, and robust solutions. One of this platform’s most potent features is Laravel Collections. In this blog post, we will dive deep into Laravel Collections, exploring their versatility and untapped potential that can have a significant impact on your Laravel projects.
The Backbone of Data Manipulation: Laravel Collections
Laravel Collections are essentials for any Laravel developer, serving as a versatile tool for data manipulation in Laravel applications. Laravel Collections act as a statistical, object-oriented layer over PHP arrays, providing an extra boost to handling and transforming data.
For instance, here's a simple Laravel Collection being created:
<?php
$collection = collect(['taylor', 'abigail', null]);
$collection->toArray(); // ['taylor', 'abigail'];
Features like these reduce the complexity of dealing with PHP arrays and provide cohesion and ease to Laravel developers, making Laravel Collections an indispensable tool in their arsenal.
Best Practices For Laravel Collections
Laravel Collections offers a wide array of methods and developers should try to utilize these instead of resorting to loops. It encourages efficient coding practices and greatly improves code readability:
<?php
$collection = collect([1, 2, 3, 4]);
$multiplied = $collection->map(fn ($item) => $item * 2);
The map()
function here eliminates the need for a loop to multiply every item by 2, ensuring a cleaner and more organized code.
Expert Tips For Laravel Collection Mastery
For developers looking to delve deeper into Laravel Collections, the tap()
helper function can prove to be immensely useful. It aids in debugging without disrupting the method chain:
<?php
$collection = collect(['laravel', 'collections', 'transform', 'data']);
$chains = $collection->sort()
->tap(fn ($collection) => Log::info('After sort', ['data' => $collection->toArray()]))
->filter(fn ($value) => strlen($value) > 5);
The use of tap()
function ensures that the intermediate result is logged without breaking the method chain. Understanding and mastering such tips can truly escalate your Laravel Collections prowess.
The Versatility and Potential of Laravel Collections
Laravel Collections offer a flexible nature that can greatly benefit developers through complex data manipulation. Laravel Collections are equipped with a multitude of versatile methods, including filtering, mapping, sorting, and reducing array data.
<?php
$collection = collect([1, 2, 3, 4]);
$filtered = $collection->filter(function ($value, $key) {
return $value > 2;
});
$filtered->all(); // [3, 4]
The filter()
method in the example effortlessly sifts through a Collection and only returns items fulfilling the defined condition.
Moreover, Laravel Collections can prove particularly effective in transforming large datasets, such as API responses:
<?php
$apiResponse = collect($apiResponse)->map(function ($item, $key) {
return [
'id' => $item['id'],
'user' => $item['name'],
'email' => $item['email'],
];
});
The map()
function here has seamlessly shaped the raw API response data, ensuring its easy processing and use.
Optimizing Performance with Laravel Collections
Laravel Collections also provides an opportunity to enhance code efficiency and optimize performance in data manipulating operations. They allow Laravel developers to generate clean, compact, and efficient code.
For example, consider the reduce()
method:
<?php
$collection = collect([1, 2, 3]);
$total = $collection->reduce(function ($carry, $value) {
return $carry + $value;
});
// Total: 6
The function aggregates the values in the collection, illustrating an efficient data processing method.
The lazy()
method, on the other hand, helps in memory consumption:
<?php
$collection = collect(range(1, 1000000));
$lazyCollection = $collection->lazy();
The lazy()
method here employs a LazyCollection
instance that saves memory usage by only holding necessary items in memory instead of the entire array.
Conclusion
Laravel Collections, equipped with impressive capabilities, offer Laravel developers the chance to write efficient, cleaner, and performance-optimized code. Through best practices, expert techniques, and understanding their versatility, developers can untap potential and achieve superior outcomes from their Laravel PHP framework usage.
If you're looking for a Laravel developer who knows the ins and outs of Laravel Collections and how best to leverage their power, consider hiring JerTheDev. With proficiency in Laravel PHP and a deep understanding of how to manipulate Laravel Collections for the best results, you can rest assured that your Laravel projects are in safe hands. Just check out the Services page for more information on what JerTheDev has to offer.
Remember, Laravel Collections are just the beginning; there's still much more to explore in the vast ocean of Laravel.
Comments
No comments